As the sun sets on 2023, I find myself reflecting on a year filled with growth, learning, and immense gratitude. This past year has been a journey like no other, all thanks to the incredible WordPress community that has supported me every step of the way. I’ve delved into the heart of WordPress, contributed to its core, and immersed myself in a world where passion and collaboration intersect. Today, I want to share my love for the WordPress community and express my excitement for the promising year that lies ahead.
A Year of Support and Learning
The WordPress community is a vibrant and diverse ecosystem, made up of passionate individuals who share a common love for open-source development, content creation, and innovation. Over the past twelve months, this community has become my second family, guiding me through challenges, celebrating victories, and fostering an environment of continuous learning.
From troubleshooting code to brainstorming creative solutions, the WordPress community has been my go-to resource for support. Whether on forums, social media, or at WordCamps, the willingness of fellow enthusiasts to lend a helping hand has been both inspiring and humbling. It’s this spirit of collaboration that makes the WordPress community truly exceptional.
A Dedication to Favorites
In the photograph above, you’ll find a visual representation of my journey and the things I hold dear. This Christmas decoration was made my wife. At the center of this dedication is the adorable Wapuu, the unofficial mascot and symbol of WordCamps. Wapuu represents not just a cute character but a sense of belonging and camaraderie within the community.
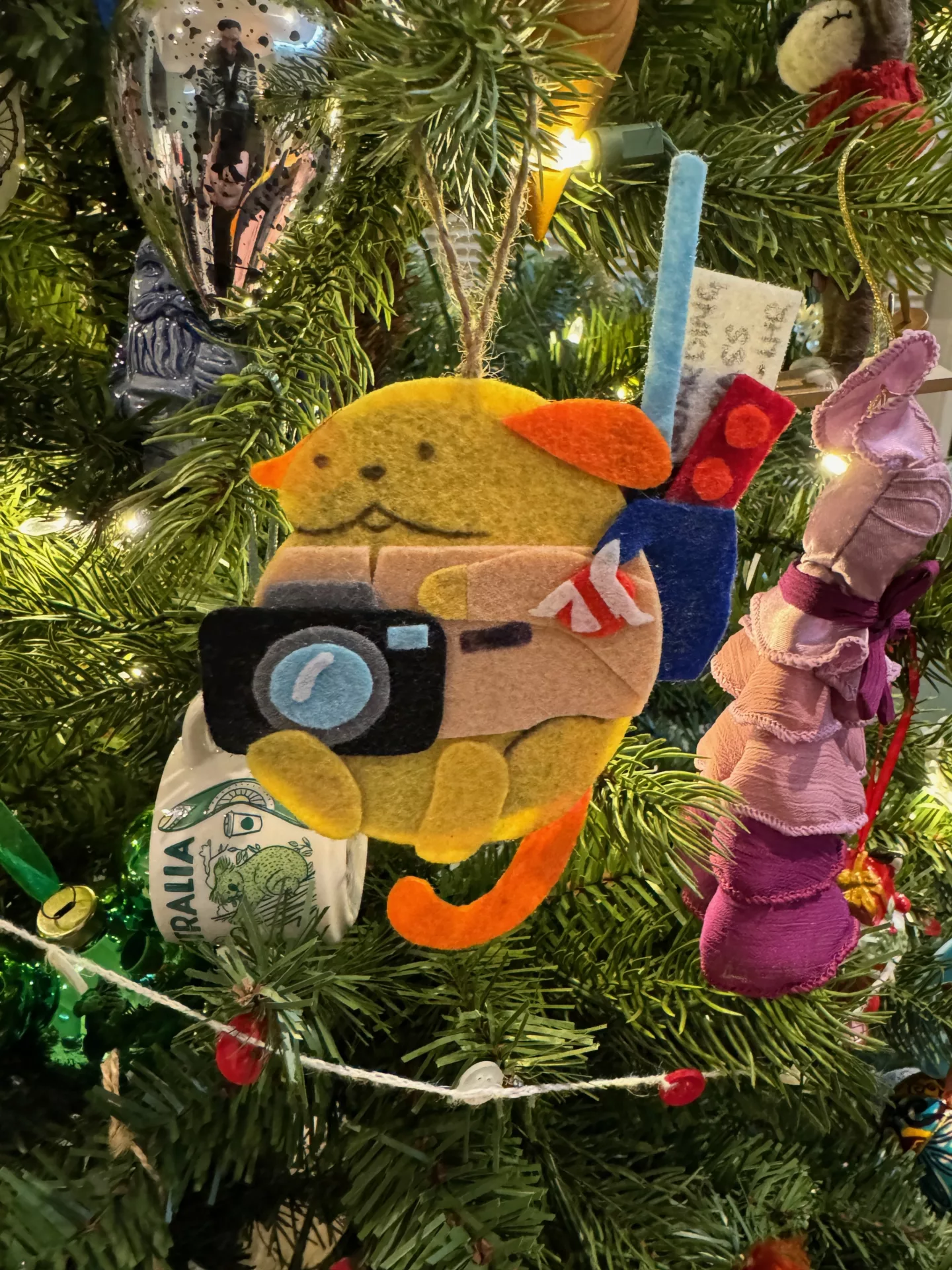
A Proud Contributor to WordPress Core
One of the highlights of my year has been contributing to the WordPress core. Working on the backbone of this powerful platform has been both challenging and immensely rewarding. It’s a privilege to be part of a global effort that shapes the future of WordPress, and I take pride in knowing that my contributions have played a small role in its evolution.
The sense of accomplishment that comes with seeing your code integrated into the core is indescribable. It’s a testament to the collaborative nature of the WordPress project, where individual efforts come together to create something truly remarkable.
Anticipating a Bigger 2024
As we stand on the brink of a new year, there’s an undeniable sense of excitement and anticipation within the WordPress community. 2023 has seen significant milestones, with updates, improvements, and a collective commitment to pushing boundaries. Looking ahead, 2024 promises to be even bigger – a year of innovation, community engagement, and continued growth.
More reading
You must be logged in to post a comment.